Domain objects overview
The following domain objects are available in the Tupertino system:
Domain Model Overview
This document provides an overview of the domain models used in the system. Each domain model plays a specific role in managing data and operations. The status column indicates the current implementation status of each domain.
Domain | Description | Status |
---|---|---|
_prisma_migrations | Handles database migrations. Direct modifications are not recommended. | Done |
casbin_rule | Manages access control rules for organizations. Modifications should be made through code. | Done |
Idempotency | Keeps track of idempotency keys for each request to ensure operations can be repeated safely. | Done |
User | Stores user profiles, including encrypted passwords. | Done |
PasswordReset | Facilitates the process for users to reset their passwords. | Done |
Organisation | Contains data related to organizations. | Done |
OrganisationInvitation | Manages invitations for new users to join an organization. | Done |
OrganisationSecret | Handles API and secret keys for an organization's applications. | Done |
CheckoutPageTemplate | Manages templates for an organization's checkout pages. | Done |
CheckoutPageTemplateOnPaymentType | Links checkout page templates to payment types within an organization. | Done |
CheckoutPage | Oversees the creation and management of checkout pages for an organization. | Done |
PaymentType | Manages the different payment types an organization can offer. | Done |
PaymentMethod | Represents a method of payment, such as bank transfers via specific systems. | Done |
OrganisationPaymentMethod | Manages the payment methods available to an organization. | Done |
OrganisationsOnUser | Manages the associations between users and organizations. | Done |
Partner | Represents a payment partner, gateway, or integrator that the system integrates with for processing payments. | Done |
Payment | Manages payment transactions for an organization, linking to partners and payment methods. | Done |
Refund | Handles refund operations for payments, allowing for multiple refunds per payment. | Done |
PartnersOnRefunds | Manages the refunds processed for an organization. | Done |
PartnersOnPayments | Oversees the payments processed for an organization. | Done |
PartnerPaymentMethod | Manages the payment methods available to a payment partner. | Done |
LedgerTransaction | Manages ledger transactions for an organization. | Done |
LedgerBalance | Keeps track of the ledger balance for an organization. | Done |
This table is intended to provide a clear and concise overview of the domain models and their current status within the system.
Relationships
The following diagram shows the relationships between objects.
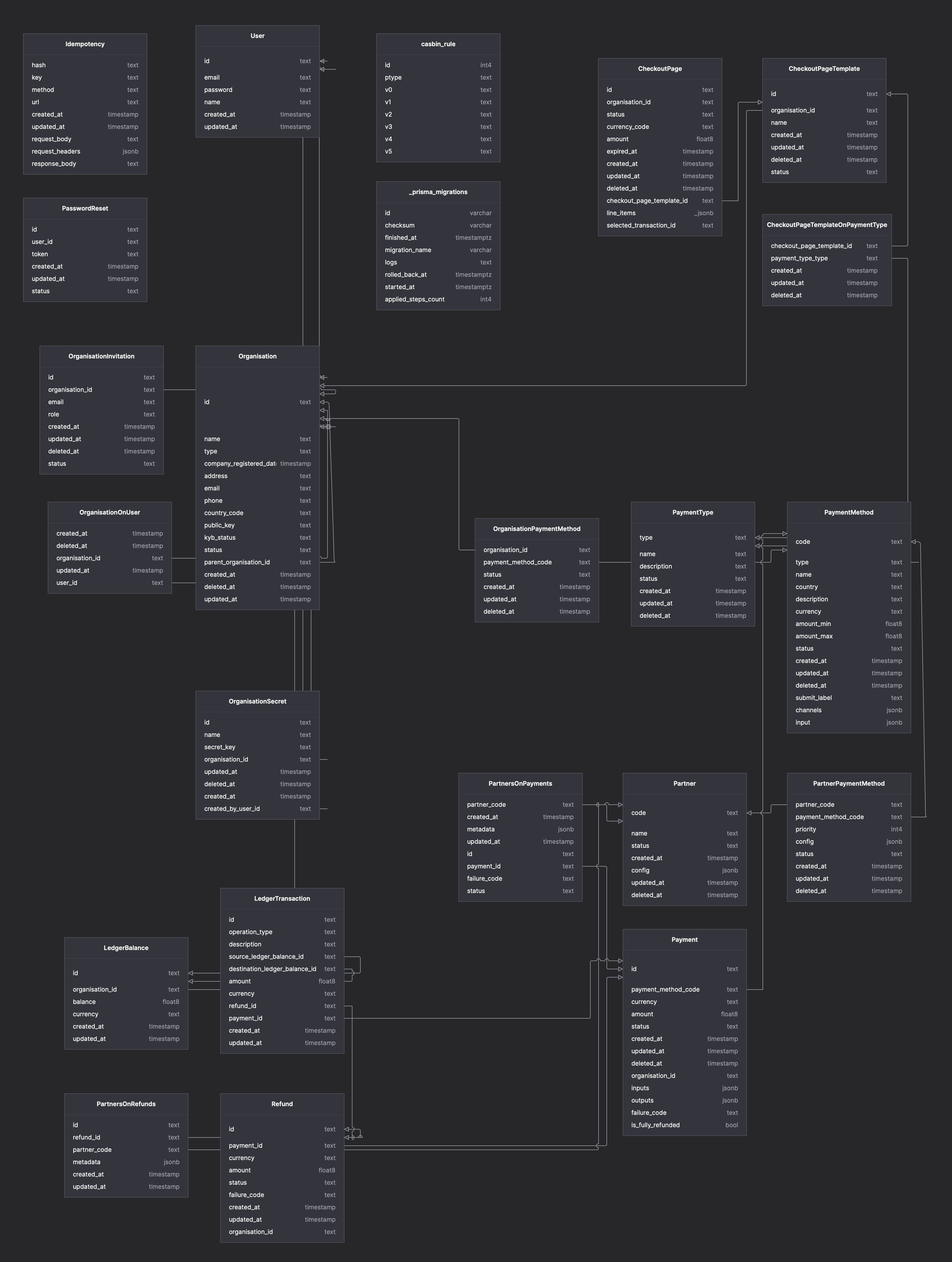
Users
The Users domain manages the information and operations for users. It includes the following:
export type User = {
id: string;
email: string;
password: string;
roles?: string[];
created_at: Date;
updated_at?: Date;
deleted_at?: Date;
};
User password resets
The User Password Resets domain manages the process of resetting a user's password. It includes the following:
type PasswordReset = {
id: string;
user_id: string;
token: string;
created_at: Date;
updated_at: Date;
status: "pending" | "accepted";
};
Organisations
The Organisations domain manages information and operations for organisations. It includes the following:
type Organisation = {
id: string;
name: string;
type: string;
company_registered_date: Date;
address: string;
email: string;
phone: string;
country_code: string;
public_key: string;
kyb_status: string;
status: string;
users: OrganisationOnUser[];
parent_organisation_id: string | null;
parent_organisation: Organisation | null;
suborganisations: Organisation[];
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
OrganisationInvitation: OrganisationInvitation[];
OrganisationPaymentMethod: OrganisationPaymentMethod[];
CheckoutPageTemplate: CheckoutPageTemplate[];
OrganisationSecret: OrganisationSecret[];
};
OrganisationInvitation
The OrganisationInvitation domain manages the process of inviting new users to an organisation. It includes the following:
type OrganisationInvitation = {
id: string;
organisation_id: string;
organisation: Organisation;
email: string;
role: string;
status: "pending" | "accepted";
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
ApiKey
The ApiKey domain manages the API keys for an organisation's applications. It includes the following:
type ApiKey = {
id: string;
organisation_id: string;
organisation: Organisation;
name: string;
key: string;
permissions: {
partners: string;
payments: string;
users: string;
reports: string;
};
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
CheckoutPageTemplate
The Checkout Page Templates domain manages the checkout page templates for an organisation. It includes the following:
type CheckoutPageTemplate = {
id: string;
organisation_id: string;
Organisation: Organisation;
name: string;
status: string;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
CheckoutPageTemplateOnPaymentTypes: CheckoutPageTemplateOnPaymentType[];
};
type CheckoutPageTemplateOnPaymentType = {
checkout_page_template_id: string;
payment_type_type: string;
CheckoutPageTemplate: CheckoutPageTemplate;
PaymentType: PaymentType;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
CheckoutPage
The Checkout Pages domain manages the checkout pages for an organisation. It includes the following:
type CheckoutPage = {
id: string;
organisation_id: string;
status: string;
selected_transaction_id: string | null;
currency_code: string;
amount: number;
line_items: any;
expired_at: Date;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
PaymentType
Payment types refer to a group of payment methods. The Payment Types domain manages the payment types available to an organisation. It includes the following:
type PaymentType = {
type: string;
name: string;
description: string;
status: string;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
payment_methods: PaymentMethod[];
CheckoutPageTemplates: CheckoutPageTemplateOnPaymentType[];
};
PaymentMethod
Payment methods refer to the specific payment methods available to an organisation. The Payment Methods domain manages the payment methods available to an organisation. It includes the following:
type PaymentMethod = {
code: string;
name: string;
type: string;
description: string;
input: any; // Replace with the actual type of the input
country: string;
currency: string;
amount_min: number;
amount_max: number;
status: string;
submit_label: string;
channels: any; // Replace with the actual type of the channels
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
Partners: PartnerPaymentMethod[];
OrganisationPaymentMethod: OrganisationPaymentMethod[];
PaymentType: PaymentType | null;
};
PaymentTransaction
PaymentTransaction manages the payment transactions for an organisation. It includes the following:
type PaymentTransaction = {
id: string;
organisation_id: string;
organisation: Organisation;
payment_method_code: string;
payment_method: PaymentMethod;
currency: string;
amount: number;
details: any; // Replace with the actual type of the details
status: string;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
Refund
Refund manages the refunds for an organisation. It includes the following:
type Refund = {
id: string;
organisation_id: string;
organisation: Organisation;
payment_transaction_id: string;
payment_transaction: PaymentTransaction;
currency: string;
amount: number;
details: any; // Replace with the actual type of the details
status: string;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};
OrganisationPaymentMethod
The Organisation's Payment Methods domain manages the payment methods available to an organisation. It includes the following:
type OrganisationPaymentMethod = {
organisation_id: string;
payment_method_code: string;
organisation: Organisation;
payment_method: PaymentMethod;
status: string;
created_at: Date;
updated_at: Date;
deleted_at: Date | null;
};